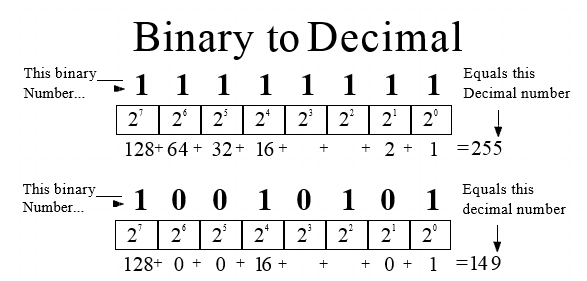
TL;DR
In the last post, we presented Binary numbers. Let’s explore Binary numbers arithmetic and interesting software testing problems that could arise. The post is aligned with the Black Box Software Testing Foundations course (BBST) designed by Rebecca Fiedler, Cem Kaner, and James Bach.
Adding
Here is how to add two 8-bit binary numbers. Remember that the number of bits is a number of boxes in which we can store our binary numbers.
23 + 18 = 41 23 = 0 0 0 1 0 1 1 1 + 18 = 0 0 0 1 0 0 1 0 41 = 0 0 1 0 1 0 0 1
Remember the following simple rules:
1 + 1 = 1 0 => here we have carry by the 1. 1 + 0 = 0 1
Overflow
The biggest number that we can store in 8 boxes (bits) is:
255 = 1 1 1 1 1 1 1 1
When we add 1 to 255, we have overflow:
255 = 1 1 1 1 1 1 1 1 1 = 0 0 0 0 0 0 0 1 256 1 0 0 0 0 0 0 0 0
We have eight carry by 1. The last carry do not have its own box, so instead of 256, we got 0! This is often called a buffer overflow attack.
To store 256, we need more bits. My Mac operating system is 64 bits operating system, which means that it operates with 64 boxes. That is enough for 256. But what about bigger numbers? We then use floating-point decimal number representation.
Signed Representation
How to sore negative numbers using binary representation? We use a leftmost bit for that. 1 is for a negative number, zero is for positive. The used algorithm is called two complements. Using 8 bits and two complements, we can store numbers from -128 to 127. Here is the calculation:
127 = 0 1 1 1 1 1 1 1 => leftmost bit is zero, which is + sign, and seven 1 are 127. -128 = 1 0 0 0 0 0 0 0 => leftmost bit is - sign.
invert every digit
= 0 1 1 1 1 1 1 1 add 1 = 1 0 0 0 0 0 0 0 => this is 128, so final result is (-) 128