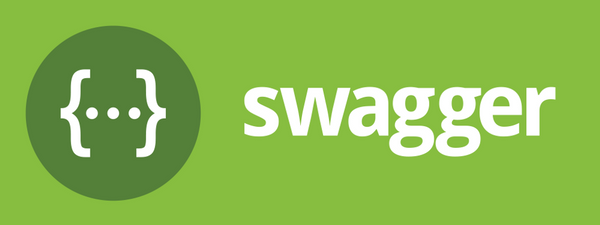
TL;DR
Swagger allows you to describe the structure of your JSON APIs so that machines can read them. Phoenix Swagger is an Elixir library that helps you to integrate Swagger into your Phoenix project. Swagger Validation enables you to reject invalid JSON requests out of the box. Setting up Phoenix Swagger validation on the production environment was not documented, so we decided to record it in this blog post.
Phoenix Swagger Validation
Configuration of Phoenix Swagger is for Phoenix >= 1.5
Phoenix Swagger validation has two components, Plug and Swagger schema parser. After you defined Swagger schema for your API endpoint, here is how you enable out of the box feature for schema validation:
router.ex
At the end of your :api
pipeline add this Phoenix Swagger plug:
plug(Validate, validation_failed_status: 422)
We decided to return HTTP
status code 422
for a JSON
request that is not according to our API endpoint Swagger schema. An additional benefit is that the user would also get a meaningful error message about what is wrong with the JSON request.
application.ex
Here you must parse your Swagger schema file:
Put as first line after the def start(_type, _args) do
PhoenixSwagger.Validator.parse_swagger_schema("priv/static/swagger.json")
Swagger schema JSON file is generated on project compilation (if you followed configuration instructions in Phoenix Swagger documentation).
This works ok for the development environment, but in production, your application start would fail with erlang :enoent
error code, because swagger.json file could not be found.
Here is the solution. You need to add production variable in config files:
dev.exs
and test.exs
config
:your_app_name, production: false
prod.exs
config :your_app_name, production: true
And in application.ex